The WooCommerce Analytics export is a powerful tool for store owners, but it has its limitations. The default CSV export file doesn’t include all the order details, probably for readability. While you can remove columns easily, there’s no graphical way to add more information.
One very good reason why you’d want to add more data is when it comes time to calculate your taxes.
Handing your accountant a CSV file with each fee, tax, total, etc., would probably save them much more time. Luckily, customizing the export to include more details is straightforward, thanks to the WooCommerce team’s addition of filters to extend the columns in the server export code.
What is a Server Export?
A server export is used when the number of orders or rows exceeds one page. In this case, the CSV file is built in the background on the server, allowing us to run our PHP logic, which we’ll discuss below. Therefore, it’s important to filter your orders so they span more than one page.
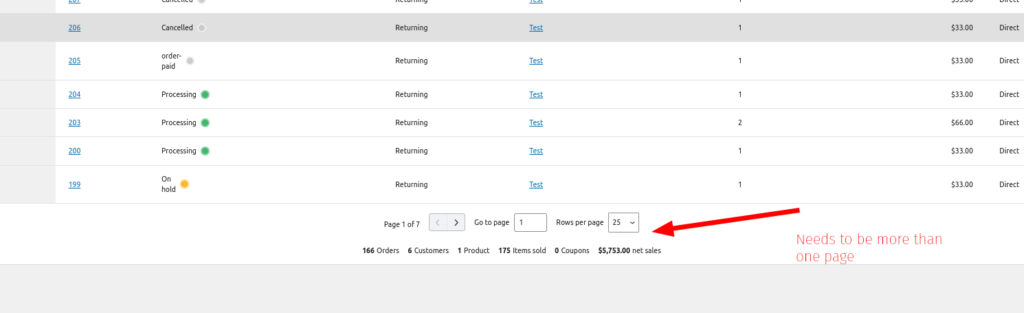
Adding Custom Columns to Your Export
Let’s dive into the code. In all of the analytics export functions, you’ll find two filters: woocommerce_report_{data}_export_columns
and woocommerce_report_{data}_prepare_export_item
. The first filter is used to add a new column, and the second to add data to that column.
The {data} can be one of:
categories, coupons, customers, downloads, orders, products
Step 1: Adding a New Column
To add a column, simply extend the existing array. Here’s an example of how to add a “Phone Number” column:
add_filter('woocommerce_report_orders_export_columns', 'get_report_export_columns');
function get_report_export_columns($export_columns)
{
$export_columns['phone_number'] = 'Phone Number';
return $export_columns;
}
This method is consistent across different types of data you may want to extend.
Step 2: Adding Data to the New Column
The next step is to add the data to the “Phone Number” column. We do this by referencing the column and assigning a value to it.
The trick here is to check the woocommerce_report_{data}_prepare_export_item
hook for the specific data you’re extending. This ensures we catch the data that provides access to an order or product object, allowing us to add the necessary details.
For example, check the order export integration here. You’ll see an exported order_number
, which we can use to get the $order
object and add our custom data.
add_filter('woocommerce_report_orders_prepare_export_item', 'prepare_export_item', 10, 2);
function prepare_export_item($export_item, $item)
{
// get the order object so we can go wild
$order = wc_get_order($export_item['order_number']);
$export_item['phone_number'] = $order->get_billing_phone();
return $export_item;
}
In the case of downloads, you get access to both the user_id and order_number
.
Piecing everything together:
add_filter('woocommerce_report_orders_export_columns', 'get_report_export_columns');
add_filter('woocommerce_report_orders_prepare_export_item', 'prepare_export_item', 10, 2);
function get_report_export_columns($export_columns)
{
$export_columns['phone_number'] = 'Phone Number';
return $export_columns;
}
function prepare_export_item($export_item, $item)
{
// get the order object so we can go wild
$order = wc_get_order($export_item['order_number']);
$export_item['phone_number'] = $order->get_billing_phone();
return $export_item;
}
In the case of downloads, you get access to both the user_id
and order_number
.
And that’s all there is to it. I hope this helps you in whatever you’re trying to achieve. : )
Need help building a custom WooCommerce/WordPress solution? Feel free to submit the contact form here for a quote.
Leave a Reply